Three ways of adding tests to the system
⭐ We’ve designed the system to make it easy for you to add your own tests and customize the Verification system to your needs. We have provided a large set of base checks, but every project has specific needs that you can tailor our system towards. If you want to add tests, there are three main ways you can do this
The IVerify interface
This is the simplest way. By implementing this interface in your MonoBehavior
or ScriptableObject
classes, you can add a custom test logic simply by implementing the Verify()
-function. See here for an overview of how this works and see below for more info on write a single test specifically.
Write a class inheriting from VerifyCheckBase
The checks that are performed on GameObjects, Assets etc are written in a modular way, allowing you to add new checks to the system easily. You see them appear as a long list when setting up a profile; each entry here is a check. In a nutshell, writing a class that inherits from the VerifyCheckBase
class and implementing one or more of its PerformCheck()
-functions does the trick. See here for more info on this!
Check attributes using VCustomValidationAttribute
This is the most complex one, but still quite straigforward. You can implement your own attribute using the VCustomValidationAttribute
or by writing a class that implements the the IVerifyAttribute
interface. See here for more info on this.
Customizing test results
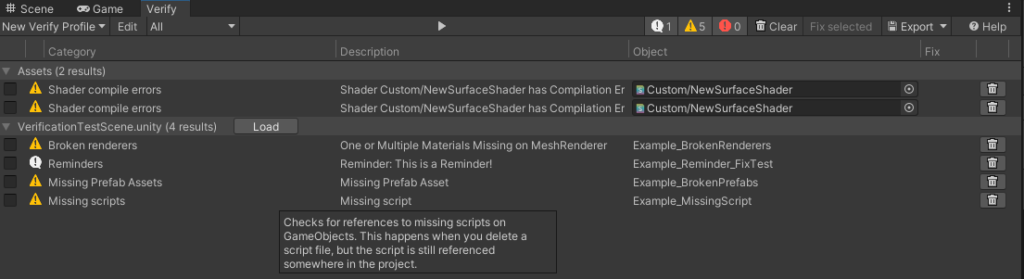
A test result in the system generally consists of the following elements:
- Category: generally the name of the check, for example “Shader compile errors” in the image above.
- Description / Error message: medium-length description of the error for added context
- Long description text: this is designed to give more info and context and appears as a tooltip when hovering over a test result
- Severity: an enum value that determines if this is an info, a warning, or an error (determines which icon to use)
- Object reference: reference to the object that failed the check, if applicable
- Action to fix it: a UnityAction that can be called to automatically fix the issue, if applicable
These info can either be passed as parameters the functions seen below, or added to a VerifyResult using the following syntax:
checker.AddFailedCheck().WithCategory(description)
.WithDescription(errorMessage)
.ForObject(obj)
.WithSeverity(m_Severity)
.WithFix(fixAction);
For example like this:
checker.AddFailedCheck().WithDescription("Val should equal 11")
.ForObject(myTestObject)
.WithFix(() => { myTestObject.val = 11; });
Or, when passed as parameters:
checker.Check(val == 11, "Val should equal 11", myTestObject, () => { myTestObject.val = 11; });
Testing for conditions
When implementing the IVerify interface or inheriting from VerifyCheckBase, you’ll reach a point where you want to add a test result to the system. This is generally done using functions from the VerifyCheckBase class like the Check() function:
public void Verify(CheckVerifyInterface checker)
{
checker.Check(myValue > 10, "Value must be larger than 10!", this);
}
If the first parameter of the Check()
-function is true, the check passes. If it is false, an entry is added to the system as a failed check using the description and object you have provided.
For convenience, there are some variants of this function:
Check(bool passes, …)
: If the first parameter of theCheck()
-function is true, the check passes. If it is false, an entry is added to the system as a failed check using the description and object you have provided.CheckNotNull(UnityEngine.Object checkedObj, …)
: If the provided object is null, the check fails and an entry is added to the system.CheckStringNotWhitespace(string checkedString, …)
: If the provided string is null or only contains whitespaces, the check fails and an entry is added to the system.CheckStringNotEmpty(string checkedString, …)
: If the provided string is empty, the check fails and an entry is added to the system.CheckElementsNotNull<T>(IEnumerable<T> checkedObj, …)
: If the provided IEnumerable (like a List, LinkedList etc) contains null values, the check fails and an entry is added to the system.
⭐ You can also use the AddFailedCheck()
-method to directly add a failed check to the system without relying on the predefined helper functions above.