As of version 1.6.0 we’ve made it much easier to animate your lights at runtime. Here’s a simple script showing how to animate light properties either using DOTween (or a similar package) or manually by setting the value every frame:
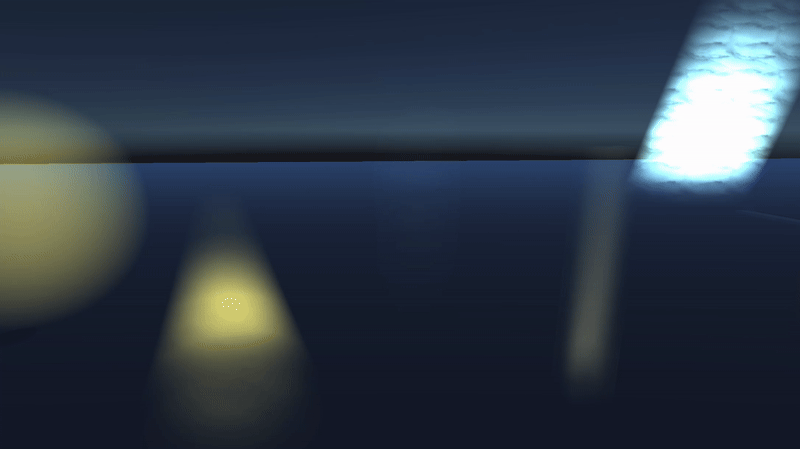
using Sparrow.VolumetricLight;
using UnityEngine;
using DG.Tweening;
public class SimpleAnimationExample : MonoBehaviour
{
[SerializeField] VolumetricLight m_Light = default;
private void Start()
{
// DOTween Example:
// DOTween.To(x => m_Light.settings.intensityMultiplier = x, 0, 3, 20f);
}
void Update()
{
m_Light.settings.intensityMultiplier = (Mathf.Sin(Time.time) + 1);
}
}
Note that these changes don’t persist after play mode ends, so you can go wild! You can also animate LightProfiles in the same way to animate multiple lights at the same time.